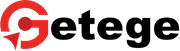
JavaScript was created by Brendan Eich in 1995 while he was working at Netscape Communications Corporation. Initially named LiveScript, it was later renamed JavaScript to capitalize on the popularity of Java at the time. Unlike Java, which is a compiled language, JavaScript is an interpreted language, meaning that code is executed line by line by the browser.
At its core, JavaScript utilizes a C-like syntax with semicolons to terminate statements. Variables in JavaScript can be declared using the var, let, or const keywords, each with its own scope rules. Data types include strings, numbers, booleans, arrays, objects, and more.
Functions are reusable blocks of code that perform a specific task. They can accept parameters and return values, making them versatile building blocks in JavaScript. Arrow functions, introduced in ES6, provide a concise syntax for writing functions.
JavaScript supports various control flow statements, including if, else if, else for conditionals, and for, while, do-while for loops. Switch statements offer an alternative for multiple branching conditions.
Arrays and objects are essential data structures in JavaScript. Arrays store collections of data, while objects represent unordered collections of key-value pairs. Array methods such as map, filter, and reduce facilitate manipulation of array elements.
The Document Object Model (DOM) is a programming interface that represents the structure of a document as a tree of nodes. JavaScript allows developers to manipulate the DOM, enabling dynamic changes to web page content and structure.
Event handling in JavaScript involves responding to user interactions such as clicks, mouse movements, and keyboard inputs. Event listeners are used to attach functions to specific events, enabling interactive behavior in web applications.
Asynchronous JavaScript enables non-blocking execution of code, crucial for tasks such as fetching data from external sources. Callbacks, promises, and async/await are mechanisms for managing asynchronous operations in JavaScript.
Error handling in JavaScript involves detecting and responding to runtime errors. Try...catch statements allow developers to gracefully handle exceptions and prevent program crashes.
ES6, short for ECMAScript 2015, introduced several new features and syntax improvements to JavaScript. Let and const provide block-scoped variable declarations, while template literals offer a convenient way to create strings with embedded expressions.
JavaScript Object Notation (JSON) is a lightweight data interchange format commonly used for transmitting data between a server and a web application. JavaScript provides built-in methods for parsing JSON strings into JavaScript objects and vice versa.
Debugging is an essential skill for JavaScript developers. Browser developer tools offer robust debugging capabilities, allowing developers to inspect and manipulate the DOM, set breakpoints, and analyze network requests.
Numerous JavaScript libraries and frameworks, such as jQuery, React, and Angular, have gained popularity for simplifying and accelerating web development. While libraries provide pre-built functions for common tasks, frameworks offer comprehensive structures for building web applications.
Adhering to best practices is crucial for writing clean, efficient, and maintainable JavaScript code. Practices such as meaningful variable names, consistent indentation, and modular code organization contribute to code readability and maintainability.
In conclusion, JavaScript is a versatile programming language that powers the dynamic and interactive aspects of modern web development. By mastering the fundamentals covered in this guide and staying abreast of new developments in the JavaScript ecosystem, developers can create engaging and responsive web experiences.